Rayminator: The Most Satisfying Way to Squash Bugs (Literally)
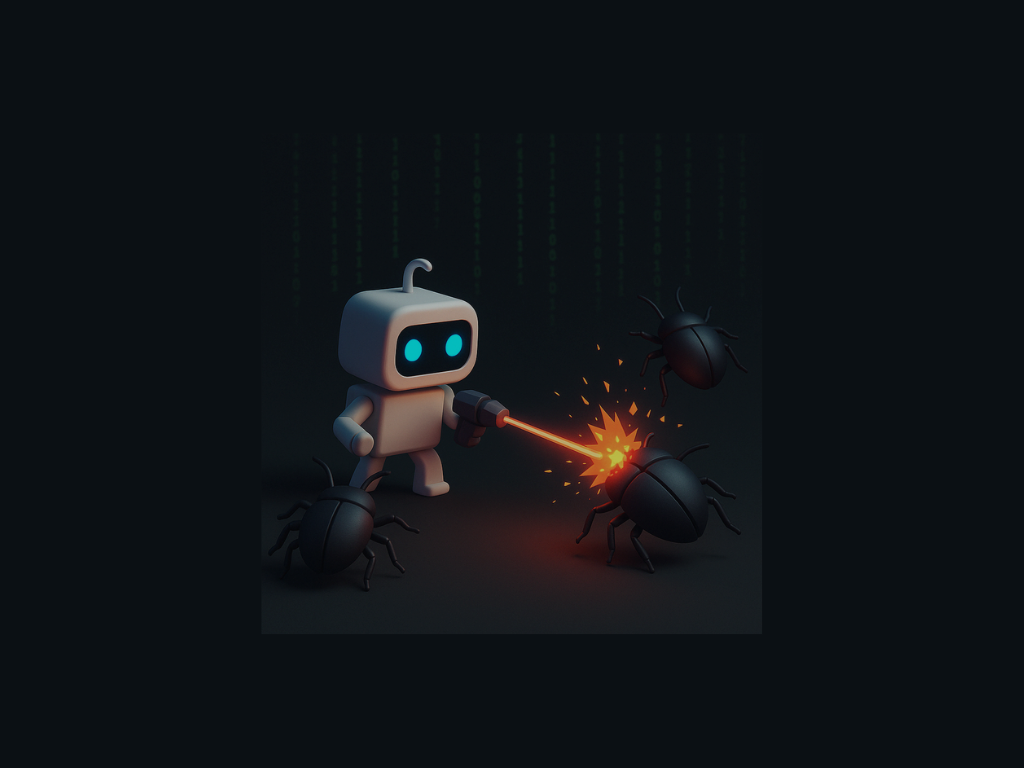
Ever had one of those days when you've been chasing the same bug for hours and just wish you could blast it into digital oblivion? Yeah, me too. That's exactly why I built Rayminator – a fun little 3D game that lets developers literally destroy bugs with laser beams.
The Birth of a Bug-Blasting Robot
The idea came to me during a particularly frustrating debugging session. I thought, "Wouldn't it be therapeutic to have some way to blast these bugs for real?" And just like that, Rayminator was born.
I wanted something that would serve two purposes:
Give me a fun way to vent my programming frustrations
Let me learn more about 3D web development in the process
Building the Bug Blaster
I decided to build this with React and Three.js (via React Three Fiber), which turned out to be a fantastic combination. The stack is pretty straightforward:
React for the UI components and state management
React Three Fiber as the Three.js wrapper for React
Drei for helper components and loaders
Some custom components for the laser effects and animations
3D models created using Meshy.ai
Speaking of models, one of the coolest parts of this project was creating the robot and bug characters. I used Meshy.ai to generate them from images, which was a game-changer. If you haven't tried it, Meshy.ai lets you convert 2D images to 3D models with impressive detail. Just upload reference images and their AI does the heavy lifting. No need to be a Blender expert!
Here's a peek at how the laser firing code works:
// Simplified version of the laser beam effect
function LaserBeam({ start, end, active, shooting }) {
// Calculate points for the laser line
const points = useMemo(() => [startV.toArray(), endV.toArray()], [startV, endV]);
// Blinking effect for extra coolness
const pulseRef = useRef(0);
useFrame(({ clock }) => {
pulseRef.current = Math.sin(clock.getElapsedTime() * (shooting ? 15 : 5)) * 0.2 + 0.8;
});
return (
<group>
<Line points={points} color="#ff3300" lineWidth={6} />
<pointLight position={endV.toArray()} intensity={5} distance={3} color="#ff3300" />
</group>
);
}
The Most Satisfying Part? Exploding Bugs!
The bug explosion effect is probably my favorite part of the project. When your laser hits a bug, it bursts into particles with a satisfying boom sound. Pure catharsis!
Each bug is essentially a 3D model that floats around on the screen. When you hit one with your laser, the explosion animation kicks in, your score goes up, and you feel just a little bit better about that production bug you've been chasing all day.
// When a bug is hit, this explosion component renders
function BugExplosion({ position, onComplete }) {
// Create particles on mount and play sound
useEffect(() => {
// Play explosion sound
window.playExplosion();
// Generate particles
// ...
}, []);
// Update particles each frame
useFrame((state, delta) => {
// Animate particles moving outward
// ...
});
// Return explosion visual elements
return (
<group position={posVec}>
{/* Particles and light effects */}
</group>
);
}
Making It Feel Right
I spent a surprising amount of time just making the game "feel good." That meant:
Getting the laser beam to look properly sci-fi with a pulsing glow
Adding Matrix-style floating characters in the background
Adding satisfying sound effects for lasers and explosions
Creating a scoring system that rewards accuracy
The game has a surprising meditative quality to it. There's something zen about methodically picking off bugs with your laser. Maybe it's the floating Matrix code in the background, or maybe it's just the satisfaction of destroying bugs without messing up your codebase.
What I Learned
Building Rayminator taught me a ton about:
Working with 3D models in the browser
Creating visual effects like lasers and explosions
Managing game state and progression
Handling mouse/keyboard input for aiming and shooting
But the most important thing I learned? Sometimes the best way to deal with frustration is to build something fun with it.
Try It Yourself!
Want to blast some bugs of your own? The project is open source and available on GitHub. Just clone the repo, run npm install
, and start blasting!
And remember – in both Rayminator and real life, debugging is all about having the right tools and keeping a cool head. Happy bug hunting!